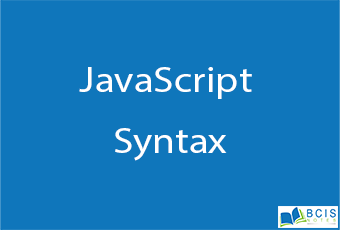
JavaScript Syntax
The syntax of JavaScript is the set of rules that define a correctly structured JavaScript program.
A JavaScript consists of JavaScript statements that are placed within the <script></script> HTML tags in a web page, or within the external JavaScript file having .js extension.
The following example shows how JavaScript statements look like:
var x = 5;
var y = 10;
var sum = x + y;
document.write(sum); // Prints variable value
Case Sensitivity in JavaScript
JavaScript is case-sensitive. This means that variables, language keywords, function names, and other identifiers must always be typed with a consistent capitalization of letters.
For example, the variable must be typed myVar, not MyVar or myvar. Similarly, the method name must be typed with the exact case, not as getElementByID().
JavaScript Comments
A comment is simply a line of text that is completely ignored by the JavaScript interpreter. Comments are usually added with the purpose of providing extra information pertaining to the source code. It will not only help you understand your code when you look after a period of time but also others who are working with you on the same project.
JavaScript support single-line as well as multi-line comments. Single-line comments begin with a double forward-slash (//), followed by the comment text. Here’s an example:
// This is my first JavaScript program
document.write(“Hello World!”);
Whereas, a multi-line comment begins with a slash and an asterisk (/*) and ends with an asterisk and slash (*/). Here’s an example of a multi-line comment.
/* This is my first program in JavaScript */
document.write(“Hello World!”);
What is a Variable?
Variables are fundamental to all programming languages. Variables are used to store data, like strings of text, numbers, etc. The data or value stored in the variables can be set, updated, and retrieved whenever needed. In general, variables are symbolic names for values.
You can create a variable with the var keyword, whereas the assignment operator (=) is used to assign value to a variable, like this: var varName = value;
var name = “Peter Parker”;
var age = 21;
var isMarried = false;
In JavaScript, variables can also be declared without having any initial values assigned to them. This is useful for variables that are supposed to hold values like user inputs.
// Declaring Variable
var userName;
// Assigning value
userName = “Clark Kent”;
Declaring Multiple Variables at Once
In addition, you can also declare multiple variables and set their initial values in a single statement. Each variable are separated by commas, as demonstrated in the following example:
// Declaring multiple Variables
var name = “Peter Parker”, age = 21, isMarried = false;
/* Longer declarations can be written to span multiple lines to improve the readability */
var name = “Peter Parker”, age = 21,
isMarried = false;
Writing Output to Browser Console
You can easily outputs a message or writes data to the browser console using the console.log() method. This is a simple, but very powerful method for generating detailed output. Here’s an example:
// Printing a simple text message console.log(“Hello World!”); // Prints: Hello World!
// Printing a variable value var x = 10;
var y = 20;
var sum = x + y; console.log(sum); // Prints: 30
Displaying Output in Alert Dialog Boxes
You can also use alert dialog boxes to display the message or output data to the user. An alert dialog box is created using the alert() method. Here’s is an example:
// Displaying a simple text message alert(“Hello World!”); // Outputs: Hello World!
// Displaying a variable value var x = 10;
var y = 20;
var sum = x + y;
alert(sum); // Outputs: 30
Writing Output to the Browser Window
You can use the document.write() method to write the content to the current document only while that document is being parsed. Here’s an example:
// Printing a simple text message document.write(“Hello World!”); // Prints: Hello World!
// Printing a variable value
var x = 10;
var y = 20;
var sum = x + y; document.write(sum); // Prints: 30
If you liked our content JavaScript Syntax, then you will also likeĀ Introduction to JavaScript
Leave a Reply