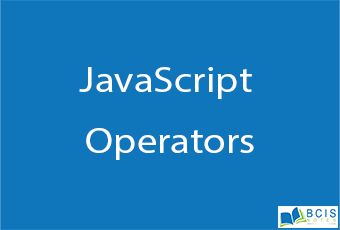
JavaScript Operators
Operators are symbols or keywords that tell the JavaScript engine to perform some sort of actions. For example, the addition (+) symbol is an operator that tells the JavaScript engine to add two variables or values, while the equal-to (==), greater-than (>), or less-than (<) symbols are the operators that tell the JavaScript engine to compare two variables or values.
Arithmetic Operator
Operator | Description | Example | Result |
+ | Addition | $a + $b | Sum of $a and $b |
– | Subtraction | $a – $b | Difference of $a and $b |
* | Multiplication | $a * $b | Product of $a and $b |
/ | Division | $a / $b | Quotient of $a and $b |
% | Modulus | $a % $b | Remainder of $a divided by $b, and so on. |
var x = 10;
var y = 5;
alert(x + y); // 0utputs: 15
alert(x – y); // 0utputs: 5
alert(x * y); // 0utputs: 50
alert(x / y); // 0utputs: 2
alert(x % y); // 0utputs: 2
JavaScript Assignment Operators
The assignment operators are used to assign values to variables.
Operator | Description | Example |
Is The Same As |
= |
Assign |
$a = $b | $a = $b |
+= |
Add and assign |
$a += $b | $a= $a + $b |
-= |
Subtract and assign |
$a -= $b | $a= $a – $b |
*= |
Multiply and assign |
$a *= $b | $a= $a * $b |
/= |
Divide and assign quotient |
$a /= $b | $a= $a / $b |
%= |
Divide and assign modulus |
$a %= $b | $a= $a % $b |
var a; // Declaring Variable
a = 20;
alert(a); // Outputs: 20
a = 20;
a += 30;
alert(a); // Outputs: 50
a = 40;
a -= 20;
alert(a); // Outputs: 20
a = 5;
a *= 15;
alert(a); // Outputs: 75
a = 40;
a /= 10;
alert(a); // Outputs: 4
a = 100;
a %= 15;
alert(a); // Outputs: 10
JavaScript String Operators
There are two operators which can also be used be for strings.
Operator | Description | Example | Result |
+ |
Concatenation |
str1 + str2 |
Concatenation of str1 and str2 |
+= |
Concatenation assignment |
str1 += str2 |
Appends the str2 to the str1 |
Example
var str1 = “Hello”;
var str2 = ” World!”;
alert(str1 + str2); // Outputs: Hello World!
str1 += str2;
alert(str1); // Outputs: Hello World!
JavaScript Incrementing and Decrementing
The increment/decrement operators are used to increment/decrement a variable’s value.
Operator |
Name |
Effect |
++a |
Pre-increment |
Increments a by one, then returns a |
a++ |
Post-increment |
Returns a, then increments a by one |
–a |
Pre-decrement |
Decrements a by one, then returns a |
a– |
Post-decrement |
Returns a, then decrements a by one |
var a; // Declaring Variable
a = 12;
alert(++a); // Outputs: 13
alert(a); // Outputs: 13
a = 12;
alert(a++); // Outputs: 12
alert(a); // Outputs: 13
a = 12;
alert(–a); // Outputs: 11
alert(a); // Outputs: 11
a = 12;
alert(a–); // Outputs: 12
alert(a); // Outputs: 11
JavaScript Logical Operators
The logical operators are typically used to combine conditional statements.
Operator |
Name |
Example |
Result |
&& |
And |
a && b |
True if both a and b are true |
|| |
Or |
a || b |
True if either a or b is true |
! |
Not |
!a |
True if a is not true |
var year = 2021;
// Leap years are divisible by 400 or by 4 but not 100
if((year % 400 == 0) || ((year % 100 != 0) && (year % 4 == 0))){
alert(year + ” is a leap year.”);
} else{
alert(year + ” is not a leap year.”); }
JavaScript Comparison Operators
The comparison operators are used to compare two values in a Boolean fashion.
Operator |
Name |
Example |
Result |
== |
Equal |
a == b |
True if a is equal to b |
=== |
Identical |
a === b |
True if a is equal to b, and they are of the same type |
!= |
Not equal |
a != b |
True if a is not equal to b |
!== |
Not identical |
a !== b |
True if a is not equal to b, or they are not of the same type |
< |
Less than |
a<b |
True if a is less than b |
> |
Greater than |
a>b |
True if a is greater than b |
>= |
Greater than or equal to |
xa>= b |
True if a is greater than or equal to b |
<= |
Less than or equal to |
xa<= b |
True if a is less than or equal to b |
var a = 35; var b = 45; var c = “35”;
alert(a == c); // Outputs: true
alert(a === c); // Outputs: false
alert(a != b); // Outputs: true
alert(a !== c); // Outputs: true
alert(a < c); // Outputs: true
alert(a > b); // Outputs: false
alert(a <= b); // Outputs: true
alert(a >= b); // Outputs: false
If you liked our content JavaScript Operators, then you will also JavaScript Data Types
Leave a Reply