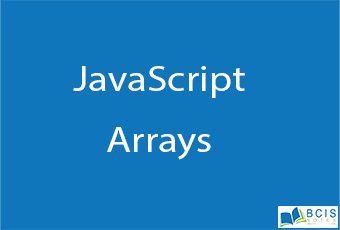
JavaScript Arrays
Arrays are complex variables that allow us to store more than one value or a group of values under a single variable name. JavaScript arrays can store any valid value, including strings, numbers, objects, functions, and even other arrays, thus making it possible to create more complex data structures such as an array of objects or an array of arrays.
Creating an Array
The simplest way to create an array in JavaScript is by enclosing a comma-separated list of values in square brackets ([]), as shown in the following syntax:
var myArray = [element0, element1, …, elementN];
An array can also be created using the Array() constructor as shown in the following syntax. However, for the sake of simplicity previous syntax is recommended.
var myArray = new Array(element0, element1, …, elementN);
Accessing the Elements of an Array
Array elements can be accessed by their index using the square bracket notation. An index is a number that represents an element’s position in an array.
Getting the Length of an Array
The length property returns the length of an array, which is the total number of elements contained in the array. Array length is always greater than the index of any of its element.
Looping Through Array Elements
Here’s the same example is rewritten using the for-of loop:
You can also iterate over the array elements using for-in loop, like this:
Adding New Elements to an Array
To add a new element at the end of an array, simply use the push() method, like this:
Similarly, to add a new element at the beginning of an array use unshift() method, like this:
Removing Elements from an Array
To remove the last element from an array you can use the pop() method. This method returns the value that was popped out.
Similarly, you can remove the first element from an array using the shift() method. This method also returns the value that was shifted out. Here’s an example:
Adding or Removing Elements at Any Position
The splice() method is a very versatile array method that allows you to add or remove elements from any index, using the syntax arr.splice(startIndex, deleteCount, elem1, …, elemN).
This method takes three parameters: the first parameter is the index at which to start splicing the array, it is required; the second parameter is the number of elements to remove (use 0 if you don’t want to remove any elements), it is optional; and the third parameter is a set of replacement elements, it is also optional.
Sorting an Array
Sorting is a common task when working with arrays. It would be used, for instance, if you want to display the city or county names in alphabetical order.
The JavaScript Array object has a built-in method sort() for sorting array elements in alphabetical order.
Reversing an Array
You can use the reverse() method to reverse the order of the elements of an array.
This method reverses an array in such a way that the first array element becomes the last, and the last array element becomes the first.
Sorting Numeric Arrays
The sort() method may produce unexpected results when it is applied to the numeric arrays (i.e. arrays containing numeric values). For instance:
To fix this sorting problem with a numeric array, you can pass a compare function, like this:
If you liked our content JavaScript Arrays, then you may also like JavaScript Conditional Statements
Leave a Reply