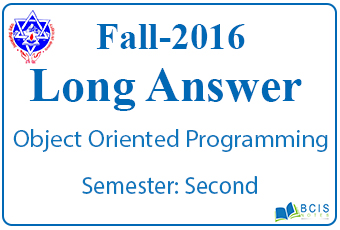
What is multithreading? Write a program to demonstrate create the thread in java.
The answer to the question “What is multithreading? Write a program to demonstrate create the thread in java.” is given below:
Multithreading in Java is a process of executing multiple threads simultaneously. A thread is a lightweight sub-process, the smallest unit of processing. Multiprocessing and multithreading, both are used to achieve multitasking. However, we use multithreading than multiprocessing because threads use a shared memory area. They don’t allocate separate memory area so saves memory, and context-switching between the threads takes less time than process.
Java Multithreading is mostly used in games, animation, etc.
Thread class provide constructors and methods to create and perform operations on a thread. Thread class extends Object class and implements Runnable interface.
class ThreadDemo implements Runnable {
Thread t;
ThreadDemo() {
t = new Thread(this, “Thread”);
System.out.println(“C thread: ” + t);
t.start();
}
public void run() {
try {
System.out.println(“C Thread”);
Thread.sleep(50);
} catch (InterruptedException e) {
System.out.println(“The C thread is interrupted.”);
}
System.out.println(“Exiting the C thread”);
}
}
public class Demo {
public static void main(String args[]) {
new ThreadDemo();
try {
System.out.println(“M Thread”);
Thread.sleep(100);
} catch (InterruptedException e) {
System.out.println(“The M thread is interrupted”);
}
System.out.println(“Exiting the M thread”);
}
}
You may also likeĀ Pokhara University, 2016 Fall Object Oriented Language
Leave a Reply