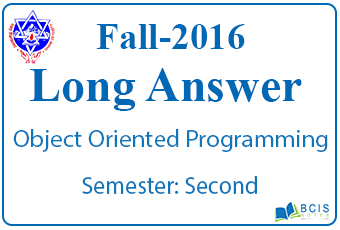
Difference between Array List and Link List? Explain the uses of the Array List with an example.
The answer to the Difference between Array List and Link List? Explain uses of Array List with an example is given below:
Parameters | Array | Linked List |
Definition | It is a collection of elements having the same data type with a common name. | It is an ordered collection of elements that are connected by links or pointers. |
Size | Fixed, once declared cannot be changed. | Variable, can be changed during run-time. |
Memory Allocation | Require Contiguous blocks of memory. | Random memory can be allocated. |
Access | Direct or randomly accessed, i.e., Specify the array index or subscript. | Sequentially accessed, i.e., Traverse starting from the first node in the list by the pointer. |
Insertion and Deletion | Relatively slow, as to insert or delete an element, other elements need to be shifted to occupy the empty space or make space. | Faster, Easier and Efficient as just linking or unlinking of the node is required. |
Searching | Binary search and Linear search | Linear search |
Extra Space | Extra space is not required. | To link nodes of the list, pointers are used which require extra space. |
Memory Utilization | Array list is inefficient | Link list is efficient |
Types | It can be single-dimensional, two dimensional or multi-dimensional. | It can be singly, doubly or circular linked list. |
import java.util.ArrayList;
class Test_ArrayList {
public static void main(String[] args) {
//Creating a generic ArrayList
ArrayList<String> arlTest = new ArrayList<String>();
//Size of arrayList
System.out.println(“Size of ArrayList at creation: ” + arlTest.size());
//Lets add some elements to it
arlTest.add(“D”);
arlTest.add(“U”);
arlTest.add(“K”);
arlTest.add(“E”);
//Recheck the size after adding elements
System.out.println(“Size of ArrayList after adding elements: ” + arlTest.size());
//Display all contents of ArrayList
System.out.println(“List of all elements: ” + arlTest);
//Remove some elements from the list
arlTest.remove(“D”);
System.out.println(“See contents after removing one element: ” + arlTest);
//Remove element by index
arlTest.remove(2);
System.out.println(“See contents after removing element by index: ” + arlTest);
//Check size after removing elements
System.out.println(“Size of arrayList after removing elements: ” + arlTest.size());
System.out.println(“List of all elements after removing elements: ” + arlTest);
//Check if the list contains “K”
System.out.println(arlTest.contains(“K”));
}
}
You may also like Pokhara University, 2016 Fall Object Oriented Language
Leave a Reply