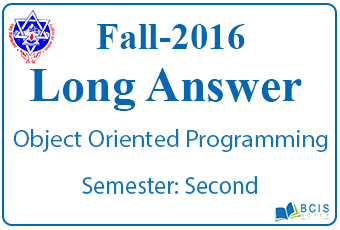
Explain the deadlock scenario in JAVA. Write a program to create your exception class.
The answer to the question “Explain the deadlock scenario in JAVA. Write a program to create your exception class.” is given below:
Deadlock in java is a part of multithreading. Deadlock can occur in a situation when a thread is waiting for an object lock, that is acquired by another thread and the second thread is waiting for an object lock that is acquired by the first thread. Since both threads are waiting for each other to release the lock, the condition is called a deadlock.
In Java, a deadlock is a situation where a minimum of two threads are holding the lock on some different resource, and both are waiting for the other’s resource to complete its task. And, none is able to leave the lock on the resource it is holding.
This usually happens when multiple threads need the same locks but obtain them in different orders. Multithreaded Programming in Java suffers from the deadlock situation because of the synchronized keyword.
It causes the executing thread to block while waiting for the lock, or monitor, associated with the specified object.
Although it is not completely possible to avoid deadlock condition, we can follow certain measures or pointers to avoid them:
- Avoid Nested Locks
- Avoid Unnecessary Locks
- Using Thread Join
import java.util.ArrayList;
import java.util.Arrays;
class CustomException extends Exception {
public CustomException(String starting) {
super(starting);
}
}
class Main {
ArrayList<String> languages = new ArrayList<>(Arrays.asList(“Java”, “Python”, “JavaScript”));
public void checkLanguage(String language) throws CustomException {
if(languages.contains(language)) {
throw new CustomException(language + ” already exists”);
}
else {
languages.add(language);
System.out.println(language + ” is added to the ArrayList”);
}
}
public static void main(String[] args) {
Main obj = new Main();
try {
obj.checkLanguage(“Swift”);
obj.checkLanguage(“Java”);
}
catch(CustomException e) {
System.out.println(“[” + e + “] Exception Occured”);
}
}
}
You may also like Pokhara University, 2016 Fall Object Oriented Language
Leave a Reply