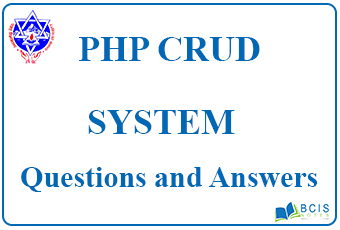
PHP CRUD System
1) What is PHP? Who created it? Write full form of MYSQL.
PHP: Hypertext Preprocessor (earlier called, Personal Home Page)
It is an HTML-embedded, server-side scripting language designed for web development.
It is server side scripting language designed for web development.
It is also used as a general purpose programming language. It was created by Rasmus Lerdorf in 1994 and appeared in the market in 1995.
2) What is XAMPP? Write differences between XAMPP, WAMP, LAMP, and MAMP.
XAMPP
The full form of XAMPP stands for Cross-platform, Apache, MariaDB(Mysql), PHP and Perl. … It is an open source platform. This includes X-OS because it works in all major operating systems like Windows, Linux, Mac etc.
The major difference between XAMPP, WAMP, LAMP, and MAMP is its operating system.
• (ie) XAMPP is for X-OS, Apache, Mysql, PHP, Perl.
• WAMP is for Windows
• LAMP is for Linux
• MAMP is for Mac OS X
XAMPP
The full form of XAMPP stands for Cross-platform, Apache, MariaDB(Mysql), PHP and Perl. It is one of the simplest and light-weight local servers that is used to test your website locally. It is an open source platform. This includes X-OS because it works in all major operating systems like Windows, Linux, Mac etc. It includes features like Filezilla, mercury mail, supporting Perl and much more. One of the main advantages is that you can perform as many testing and update the content in your website testing locally. Since it is an open source, you can easily download and install in your system. You can perform a number of testing installing it once.
WAMP
The full form of WAMP stands for Windows, Apache, Mysql, and PHP. This server works only on Windows operating system. It is an open source platform and uses the Apache web server. It also uses the MySQL relational database management system and PHP object-oriented scripting language. The important part of WAMP is Apache that is used to run a web server on windows. By run+ning this local server on windows, the web developer can tes¬t their web pages without publishing them lively. With this local server, you can test dynamic websites without publishing it on the live web server. It is easy to use and code with PHP. It is available for both 32 bit and 64-bit system.
LAMP
The full form of LAMP stands for Linux, Apache, Mysql, and PHP. It is an open source platform and works on the Linux operating system. It uses Apache web server, MySQL relational database management system, and PHP object-oriented scripting language. Since this platform has four layers, it can also be called a LAMP stack. It is highly secured working with Linux OS. The LAMP is easy to code with PHP. It is a cheap and ubiquitous hosting platform. Instead of only serving static HTML pages, a LAMP server can generate dynamic web pages that run PHP code and load data from a MySQL database.
MAMP
The full form of MAMP stands for Mac, Apache, Mysql, and PHP. MAMP is an open source platform and it works on Mac operating system. As the above local server, MAMP uses Apache web server, Mysql relational database management system, and PHP object-oriented language. It gives you all the tools that you run WordPress on your machine, for the purpose of development and testing. You can install this in Mac or Windows-based PC.
3) Write all decision making statement? Write any program to explain if else statement.
Decision making
• if…else statement − use this statement if you want to execute a set of code when a condition is true and another if the condition is not true
• elseif statement − is used with the if…else statement to execute a set of code if one of the several condition is true
• switch statement − is used if you want to select one of many blocks of code to be executed, use the Switch statement. The switch statement is used to avoid long blocks of if..elseif..else code.
#Looping statement
• for − loops through a block of code a specified number of times.
• while − loops through a block of code if and as long as a specified condition is true.
• do…while − loops through a block of code once, and then repeats the loop as long as a special condition is true.
• foreach − loops through a block of code for each element in an array.
#include
It include files and if the files is not found, it still loads the remaining code of file.
#require
It include files and if the files is not found, it doesnot loads the remaining code of file.
12) What is database? Gives examples of database.
Database
A database is an organized collection of structured information, or data, typically stored electronically in a computer system.
Types of database
Oracle, IBM DB2, Microsoft SQL(mssql) Server, Sybase, MySQL/MariaDB, PostgreSQL,msaccess
e.g.
– Banking software
– Airlines systems
– University systems
– Telecommunication systems
– News Website
– Telephone diary
Example = Banks must have systems for keeping track of customers and their accounts.
13) What is DBMS? Give examples of DBMS.
DBMS(Database management System)
A database management system is a collection of programs that enables you to store, modify, and extract information from database.
Or
A DBMS is a computer software designed to manage a database and run operation according to user requirement.
It also allows the user to add, change, and delete data, sort and retrieve them from the database and create forms and reports using the data in database.
Some popular DBMS software packages are MS-Access, FoxPro, dBase, INGRES, MySQL, SQL Server, Oracle Database, Sybase, and Informix etc.
14) Write few application area of database.
The applications or (used area) of database are (imp)
-Banking software
-Airlines systems
-University systems
-Telecommunication systems
-News Website
-Payroll
-Orders
-Ticket Reservation systems
-Accounting
-Medical records
-Inventory
-Shipping
-Security
-Mailing
15) what is CRUD?
CRUD is a system used in overall for convenience and easiness as database. In simple words, in computer programming, create, read, update, and delete are the four basic functions of persistent storage.
C=CREATE
R=READ (SELECT)
U=UPDATE
D=DELETE
What does MySQL mean?
MySQL is the most popular Open Source SQL database management system. It is named after co-founder Monty Widenius’s daughter, My. The name of Dolphin in MySQL logo is “Sakila”. The official way to pronounce “MySQL” is “My S-Q-L” (not “my sequel”). The SQL part of “MySQL” stands for “Structured Query Language.” MySQL is developed, distributed, and supported by Oracle Corporation.
Mysql
three important function
mysqli_connect($connect);
mysqli_query($connect,$query);
mysqli_close($conn);
Q) Write the relationship between a database and a table.
Answer: A database contains multiple tables whereas, a table cannot exist outside a database.
Q) Write difference between record and field in database.
Record (row) Field (column)
It is a collection of data items, which represent a complete unit of information about a thing or a person. It is an area within the record reserved for a specific piece of data.
A record refers to a row in the table. A field refers to a column in the table.
Record is also known as tuple. Field is also known as attribute.
e.g. if Employee is a table, then entire information of an employee is called a record. e.g. if Empolyee is a table, then empld, empName, department, salary are the fields.
Write total php code to create a table “contact” inside database “contact_system”
<?php
$servername=”localhost”;
$username=”root”;
$password=””;
$databasename=”contact_system”;
$conn=mysqli_connect($servername,$username,$password,$databasename);
$query=”CREATEDATABASEcontact_system”;
$query1=”CREATETABLEcontact(
idINT AUTO_INCREMENT PRIMARYKEY,
nameVARCHAR(100) NOTNULL,
phoneVARCHAR(100) NOTNULL
)”;
$query2=”CREATETABLE `contact_system`.`Co` ( `id` INTNOTNULL AUTO_INCREMENT , `name` VARCHAR(100) NOTNULL , `phone` VARCHAR(100) NOTNULL , PRIMARYKEY (`id`)) ENGINE =InnoDB;”;
$runquery=mysqli_query($conn,$query2);
mysqli_close($conn);
?>
Write total php code to insert data in table using a html form.
<?php
$servername=”localhost”;
$username=”root”;
$password=””;
$databasename=”contact_system”;
$conn=mysqli_connect($servername,$username,$password,$databasename);
if(isset($_GET[‘submit’]))
{
$name= $_GET[‘name’];
$phone=$_GET[‘phone’];
echo$name;
echo$phone;
$query3=”INSERTINTO contact (id,name,phone) VALUES (”,’$name’,’$phone’)”;
$runquery=mysqli_query($conn,$query3);
}
?>
<html>
<head>
<title>Document</title>
</head>
<body>
<formaction=””method=”get”>
name<inputtype=”text”name=”name”>
phone<inputtype=”text”name=”phone”>
<inputtype=”submit”name=”submit”>
</form>
</body>
</html>
<?php
mysqli_close($conn);
?>
Write total php code to display data from database table to html table.
<?php
$servername=”localhost”;
$username=”root”;
$password=””;
$databasename=”contact_system”;
$conn=mysqli_connect($servername,$username,$password,$databasename);
?>
<!DOCTYPEhtml>
<htmllang=”en”>
<head>
<metacharset=”UTF-8″>
<metaname=”viewport”content=”width=device-width, initial-scale=1.0″>
<metahttp-equiv=”X-UA-Compatible”content=”ie=edge”>
<title>Document</title>
</head>
<body>
<tableborder=”1px”>
<tr><td>id</td><td>name</td><td>phone</td><td>edit</td><td>delete</td></tr>
<?php
$query4=”SELECT*FROM contact”;
$runquery=mysqli_query($conn,$query4);
$i=1;
while($result=mysqli_fetch_array($runquery))
{
?>
<tr><td><?phpecho$i; ?></td>
<td><?phpecho$result[‘name’]; ?></td>
<td><?phpecho$result[‘phone’]; ?></td>
<td><ahref=”editform.php?e_id=<?phpecho$result[‘id’]; ?>”>edit</a></td>
<td><ahref=”form.php?d_id=<?phpecho$result[‘id’]; ?>”>delete</a></td></tr>
<?php
$i=$i+1;
}
?>
</table>
</body>
</html>
<?php
mysqli_close($conn);
?>
Further topics to study in php:
Data and Information and File
Data is collection of raw facts and figures, Data consists of letter, sound, image or video within the computer.
The Processed data is known as information. Using a database program or software, they are converted into the meaningful result called information.
File is a collection of electronic information stored in computer.
Database
A database is a collection of interrelated data. It refers to an organized collection of data stored on a computer in such a way that its content can easily be accessed, updated and queried upon with the help of a software program.
A collection of related information organized as tables is known as database.
Component of database
1) Data
2) Software
3) Hardware
4) Users:
It is the person, who needs information from the database to carry out its primary business responsibilities.
5) DBA (Database Administrator)
A person, who is responsible for managing and handling the overall database management system is called DBA
6) Application programmers
The person, who write programs to interact and manipulate the database is called application programmers.
Advantages of database or DBMS (imp)
-database helps to save the data in organized way.
-it reduces data redundancy on the file.
-Easy for adding, editing and removing of data.
-it has very high data integrity (reliability).
-Retrieval of information quickly.
-Sorting, selecting data that satisfies certain criteria (filtering).
-Produce the report in some standardized and readable format.
-Database protects from unauthorized access and use of data.
-It saves data from being lost.
Disadvantages of database or DBMS
-complex database system
-professional trained person is need to handle database
-maintenance cost is more
-Costly and time-consuming procedure
-Additional hardware and software may be required.
Features of DBMS: (imp)
– Easy to access data
– Ease to modify data
– Delete existing data
– Sorting and indexing of data
– Easy queries in data
– Print the formatted reports, labels etc
– Linking between two or more databases.
Any four database management systems are as follows:
i. Relational Database Management System
ii. Hierarchical Database Management System
iii. Network Database Management System
iv. Object Oriented Database Management System
Indexing
Indexing is a way of sorting(arranging) a number of records on multiple fields.
The indexing is a type of data structure. It is used to locate and access the data in a database table quickly.
Sorting
The process of arranging all the record fields is known as sorting
Filtering
The process of viewing required record of a table that matches the specified criteria is known as filtering.
Tuple
A record row in database is called tuple. In another word the row wise records collectively known as tuples.
Key field
The key is defined as the column or the set of columns of the database table which is used to identify each record uniquely.
Types of field keys: (imp)
1) Primary key
2) Compound key or composite key
3) Foreign key
4) Alternative key
5) Candidate key
6) Super key
Primary key (imp)
Primary key is a special field in the table that uniquely identifies each record from the database. The primary key does not accept duplicate value for a field and it does not allow a user to leave the field blank or null. It allows both number and text in its field.
The importance of primary key (imp)
a) To identify each record of table uniquely within very short time.
b) To reduce and control duplication of the record in a table
c) To set the relationship between tables.
Compound key or composite key
A table having more than one field with primary key field is called compound key or composite key.
The compound key are used when one primary key is not able to unique identify the record.
Foreign key
A Primary key field of one table also appears in other tables then the particular field is called foreign key. It is linking pin between two pin is called foreign key. It shows the relation between two tables.
Alternative key
The fields other than primary key field is called is called alternative key or secondary key.
Candidate key
The set of all attributes which uniquely identify each row of a relation are known as candidate keys.
e.g. column studentid and combination of firstname, lastname, phone works as candidate keys.
Super key
A super key is a combination of columns that uniquely identifies any row within a relational database management system (RDBMS) table.
Examples of superkeys are as follows:
1) ID + Name+Birthdate
Query
Query is a way of extracting information from a database, usually by using certain criteria or requesting certain data. It is request for database records that fit specified criteria.
Uses of query
i. A query can count the amount of records that meet the certain criteria.
ii. It can also be used to extract data to a separate table or delete data, change data and many other things.
Apache
Apache is the most widely used web server software. Developed and maintained by Apache Software Foundation, Apache is an open source software available for free. It runs on 67% of all webservers in the world. It is fast, reliable, and secure. It can be highly customized to meet the needs of many different environments by using extensions and modules. Most WordPress hosting providers use Apache as their web server software. However, WordPress can run on other web server software as well.
Web server
A web server is a computer that runs websites.
#to view detail of php version
Phpinfo();
#php configuration file
Php.ini
Location:xamp/php/php.ini
#language construct
Echo “hello”
Print “world”
Print is old method not used now a days.
#use of single quote vs double quote
echo'<input type=”text” name=”name”>’;
echo'<input type=\”text\” name=\”name\”>’;
You may also like What is PHP
Leave a Reply