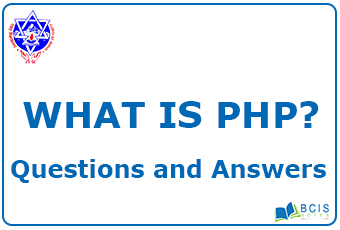
1) Answer the following question. (2 x 10 = 20 marks)
a) What is PHP? Who created it? Write a full form of MYSQL.
PHP is a server scripting language, and a powerful tool for making dynamic and interactive Web pages. PHP is a widely-used, free, and efficient alternative to competitors such as Microsoft’s ASP. It was originally created by Rasmus Lerdorf in 1994; the PHP reference implementation is now produced by The PHP Group.MYSQL name is a combination of “My”, the name of co-founder Michael Widenius’s daughter, and “SQL”, the abbreviation for Structured Query Language.
b) What is XAMPP? Write differences between XAMPP, WAMP, LAMP, and MAMP.
XAMPP is a free and open-source cross-platform web server solution stack package developed by Apache Friends, consisting mainly of the Apache HTTP Server, MariaDB database, and interpreters for scripts written in the PHP and Perl programming languages.
Difference between XAMPP, WAMP, LAMP, and MAMP as follow.
WAMP
WAMP is used for Windows operating system only.
Its full form is Windows, Apache, MySQL, and PHP
It is an open-source platform.
It uses the Apache webserver.
The relational database management system for WAMP is MySQL.
PHP (Hypertext Preprocessor) is the object-oriented scripting language.
WAMP is very easy to set up and configure.
LAMP
LAMP is used for Linux operating system only.
Its full form is Linux, Apache, MySQL, and PHP
It is also an open-source platform.
It uses the Apache webserver.
The relational database management system for LAMP is MySQL.
PHP (Hypertext Preprocessor) is the object-oriented scripting language.
MAMP
MAMP is used for Mac operating system only.
Its full form is MAC, Apache, MySQL, and PHP
It is also an open-source platform.
It uses the Apache webserver.
The relational database management system for LAMP is MySQL.
PHP (Hypertext Preprocessor) is the object-oriented scripting language.
XAMPP
XAMPP can be used for any operating system.
Its full form is x-os, Apache, MariaDB, PHP, and Perl.
X-os implies that it is a cross-platform application and can be used for any operating system.
It is a free, open-source, cross-platform web server solution pack and is developed by Apache Friends.
XAMPP comes with extra features like supporting Perl, Filezilla, mercury mail and some other scripts.
It is simple, lightweight and easy to use for developers in creating a local webserver.
c) Write all the decision-making statements? Write any program to explain the if-else statement.
In PHP we have the following conditional statements:
- if statement – executes some code if one condition is true
- if…else statement – executes some code if a condition is true and another code if that condition is false
- if…elseif…else statement – executes different codes for more than two conditions
- switch statement – selects one of many blocks of code to be executed
Example:
<?php
$t = date(“H”);
if ($t < “20”) {
echo “Have a good day!”;
}
?>
d) What is looping? What is nested looping?
Often when you write code, you want the same block of code to run over and over again a certain number of times. So, instead of adding several almost equal code-lines in a script, we can use loops.
a nested loop is a loop within a loop, an inner loop within the body of an outer one.
e) Write all the looping statements. Write any PHP code to explain for a loop.
while – loops through a block of code as long as the specified condition is true
do…while – loops through a block of code once, and then repeats the loop as long as the specified condition is true
for – loops through a block of code a specified number of times
foreach – loops through a block of code for each element in an array
Example:
<?php
for($i=1;$i<=10;$i++)
{
echo $i.”<br>”;
}
?>
f) What is the operator? Write any four operator types. Write one example of each.
Operators are used to performing operations on variables and values.
PHP divides the operators into the following groups:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Increment/Decrement operators
Example:
Assignment operators
operator | Name | Example | Result | Show it |
---|---|---|---|---|
+ | Addition | $x + $y | Sum of $x and $y |
Arithmetic operators
Assignment | Same as… | Description | Show it |
---|---|---|---|
x = y | x = y | The left operand gets set to the value of the expression on the right |
Comparison operators
Operator | Name | Example | Result | Show it |
---|---|---|---|---|
== | Equal | $x == $y | Returns true if $x is equal to $y |
Increment/Decrement operators
Operator | Name | Description | Show it |
---|---|---|---|
++$x | Pre-increment | Increments $x by one, then returns $x |
h) What is an array in PHP? Write any two ways to define an array in PHP.
Arrays in PHP is a type of data structure that allows us to store multiple elements of similar data type under a single variable thereby saving us the effort of creating a different variable for every data. They are :
- by indexing
- by associative array(relational)
<?php
$a=5;
$b=array(17,5, “ram”);
$c[0]=67;
$d[1]=”apple”;
$r=[1,”hary”,3];
echo $r[2].”<br>”;
//echo $a.”<br>”;
//echo $b;
//echo $b[2];
// indexing by using index//
for($i=0; $i<count($b); $i++)
{
echo $b[$i].”<br>”;
}
//acesing index through loop
?>
i) Write three types of an array and write PHP code to explain any one of them.
In PHP, there are three types of arrays:
- Indexed arrays – Arrays with a numeric index
- Associative arrays – Arrays with named keys
- Multidimensional arrays – Arrays containing one or more arrays
Example:
<?php
$cars = array(“Volvo”, “BMW”, “Toyota”);
echo count($cars);
?>
j) What is the function? What is the library or built-in function?
PHP functions are similar to other programming languages. A function is a piece of code that takes one more input in the form of parameter and does some processing and returns a value. Example:fopen() and fread()
Library or built-in function are inbuilt functions which are grouped together and placed in a commonplace called library. Also It is that which is built into an application and can be accessed by end-users.
OR
We need not to declare and define these functions as they are already written in the libraries such as iostream, cmath etc. We can directly call them when we need.
k) What is a user-defined function? Write different types of user-defined functions and write PHP code to explain any one function.
The functions that we declare and write in our programs are user-defined functions. You can create your own custom functions.
Types of it are:
- Function with no arguments and no return value.
- Function with no arguments and a return value.
- Function with arguments and no return value.
- Function with arguments and a return value.
PHP code
<?php
function writeMsg() {
echo “Hello world!”;
}
writeMsg(); // call the function
?>
l) Write the difference between include and require in PHP.
The difference between include and require arises when the file being included cannot be found: include will emit a warning (E_WARNING) and the script will continue, whereas require will emit a fatal error (E_COMPILE_ERROR) and halt the script. If the file being included is critical to the rest of the script running correctly then you need to use require.
m) What is the difference between local and global variables in PHP? Write PHP code to define the local variables into a global variable.
Parameter | Local | Global |
Scope | It is declared inside a function. | It is declared outside the function. |
Value | If it is not initialized, a garbage value is stored | If it is not initialized zero is stored as default. |
Lifetime | It is created when the function starts execution and lost when the functions terminate. | It is created before the program’s global execution starts and lost when the program terminates. |
Data sharing | Data sharing is not possible as data of the local variable can be accessed by only one function. | Data sharing is possible as multiple functions can access the same global variable. |
<?php
$l=5;
$b=55;
/*function area(&$l,&$b)
{
$b=$l*$b;
echo $b.”<br>”;
}
area($l,$b);
echo $b;
*/
function area()
{
global $l;
global $b;
$b=$l*$b;
echo $b.”<br>”;
}
area();
?>
n) What is the variable in PHP? Write a few lines of PHP code and assign values to a variable.
Variables are used to store data, like string of text, numbers, etc. … PHP automatically converts the variable to the correct data type, depending on its value. After declaring a variable it can be reused throughout the code.
<?php
$a=5;
$b=10;
$c=”hello”;
$d=”5.5″;
echo $a+$b.”<br>”;
echo $a+$d;
?>
o) What is constant in PHP? Write a few PHP codes to define a constant.
A constant is an identifier (name) for a simple value. The value cannot be changed during the script.
<?php
define(“GREETING”, “Welcome to Bcis notes”);
echo GREETING;
?>
p) What is a nested loop? Write PHP code to explain for each loop.
Loop inside a loop is called a nested loop.
<?php
for($i=1;$i<=5; $i++)
{for($j=1; $j<=$i; $j++)
{
echo $j;
}
echo “<br>”;
}
?>
q) What is a database? Gives examples of the database.
Databases are useful for storing information categorically. SQL Server, Oracle Database, Sybase, Informix, and MySQL are examples.
r) What is DBMS? Give examples of DBMS.
DBMS is a software system that uses a standard method of cataloging, retrieving, and running queries on data or that software that manages the database. MySQL, PostgreSQL, Microsoft Access, SQL Server, FileMaker, Oracle, RDBMS, dBASE, Clipper, and FoxPro are examples.
s) What is a table, record(row), field(column), query, sorting, index?
A database table has its own unique name and consists of columns and rows. It is the whole detail of any database.
Records(row) refers to the complete detail of a particular database, it is in row form. We can sort easily by looking at the rows.
Field(column) holds the categorization of a database like a number, id, name, etc.
A query is a request for data or information from a database table or combination of tables.
Sorting refers to the process that involves arranging the data into some meaningful order to make it easier to understand.
Index refers to the number by which we can filter from many data.
t) Write a few application areas of the database.
- Hospital
- Banking center
- Railway
- Library management system, etc
u) What is the statement used to display output in PHP? What is the statement used in PHP to show what kind of value is stored in a variable? What is the statement used in PHP to show output in a readable format?
echo and print are used to display output.
var_char() is used to show what kind of value is stored in a variable.
print_r() is the statement used in PHP to show output in a readable format.
v) give an example in PHP to
1) embedding PHP inside HTML
<?php
$time=”goodevening kdnjd;<i>ddad</i>idjaiodjiodueioudasdcewyd8owbueaybduey8pe8pde8
<h1>Nepal is a beutiful country. </h1> <p>nxksjisjisnjsushusih
sssus
jdchuhu
bjcdhu
shishish
sxhsx </p>”;
?>
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<meta http-equiv=”X-UA-Compatible” content=”ie=edge”>
<title>Document</title>
</head>
<body>
<h1><?php echo $time ?></h1>
</body>
</html>
2)embedding HTML inside PHP
<?php
$a=5;
$b=10;
$c=”hello”;
$d=”world”;
echo $a.$b.”<br>”;
echo $b;
echo “<h1>hello</h1>”;
echo $c.” “.$d;
?>
You may also like Sociology.
Leave a Reply