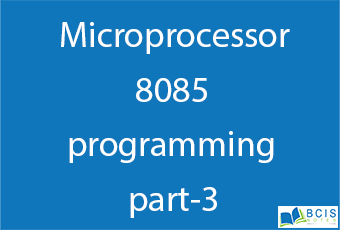
Microprocessor 8085 programming (Memory Location)
1. An 8-bit binary number is stored in memory location 1120H. WAP to store ASCII codes of these binary digits (0 to F) in location 1160H and 1161H.
LXI SP, 2000H CODE: CPI 0AH
LXI H, 1120H JC L1
LXI D, 1160H ADD 07H
MOV A, M L1: ADD 30H
ANI F0H RET
RRC
RRC
RRC
RRC
CALL CODE
STAX D
INX D
MOV A, M
ANI 0FH
CALL CODE
STAX D
HLT
2. WAP to convert ASCII at location 1040H to binary and store at location 1050H.
LXI SP, 2000H CODE: CPI 40H
LXI H, 1040H JC L1
LXI D, 1050H SUB 07H
MOV A, M L1: SUB 30H
ANI F0H RET
RRC
RRC
RRC
RRC
CALL CODE
STAX D
INX D
MOV A, M
ANI 0FH
CALL CODE
STAX D
HLT
3. A set of three packed BCD numbers are stored in memory locations starting at 1150H. The seven-segment codes of digits 0 to 9 for a common cathode LED are stored in memory locations starting at 1170H and the output buffer memory is reserved at 1190H. WAP to unpack the BCD number and select an appropriate seven-segment code for each digit. The codes should be stored in an output buffer memory.
LXI SP, 2999H CODE: PUSH H
LXI H, 1150H LXI H, 1170H
MVI D, 03H ADD L
LXI B, 1190H MOV L, A
NEXT: MOV A, M MOV A, M
ANI F0H STAX B
RRC POP H
RRC RET
RRC
RRC
CALL CODE
INX B
MOV A, M
ANI 0FH
CALL CODE
INX B
INX H
DCR D
JNZ NEXT
HLT
4. A multiplicand is stored in memory location 1150H and a multiplier is stored in location 1151H. WAP to multiply these numbers and store results from 1160H.
MVI B, 08H
MVI D, 00H
LXI H, 1150H
MOV A, M
MOV E, A
LXI H, 1151H
MOV A, M
L2: RAR
JNC L1
LXI H, 0000H
DAD D
L1: XCHG
DAD H
XCHG
DCR B
LNZ L2
HLT
5. A set of ten packed BCD numbers is stored in the memory location starting at 1150H. WAP to add these numbers to BCD. If the carry is generated save it in register B and adjust it for BCD.
The final sum is less than 9999BCD.
LXI SP, 2000H
LXI H, 1150H
MVI C, 0AH
XRA A
MOV B, A
L1: CALL ADD
INX H
DCR C
JNZ L1
HLT
ADD: ADD M
DAA
RNC
MOV D, A
MOV A, B
ADI 01H
DAA
MOV B, A
MOV A, D
RET
HLT
6. A dividend is stored in memory location 2020H and a divisor is stored in 2021H. WAP to divide these numbers and store quotient and remainder from 2040H.
MVI C, 00H
LXI H, 2021H
MOV A, M
MOV D, A
DCX H
MOV B, M
L2: MOV A, B
SUB D
JC L1
MOV B, A
INR C
JMP L2
L1: MOV L, C
MOV H, B
SHLD 2040H
HLT
7. Write a program for 8085 to convert and copy the ten lower case ASCII codes to upper case from memory location 9050H to 90A0H if any, otherwise copy as they are. Assume there are fifty codes in the source memory. [ Note: ASCII code for A=65 … Z=90, a=97 … z=122]. [2063 Kartik]
LXI H, 9050H
LXI D, 90A0H
MVI C, 32H
L2: MOV A, M
CPI 60H
JC L1
SUI 20H
L1: STAX D
DCR C
JNZ L2
HLT
8. Write a program for 8085 to add ten 16-bit BCD numbers from location 4050H and store 24- bit BCD result at the end of the ten given numbers. [2062 Chaitra]
LXI B, 4050H; Starting location of the 16-bit BCD Numbers
LXI D, 0000H
LXI H, 0000H
MVI A, 00H
L2: LDAX B
ADD L
INX B
LDAX B
ADC H
JNC L1
INR E
L1: INX B
MOV A, C
CPI 0AH
JC L2
MOV A, L
STAX B
INX B
MOV A, H
STAX B
INX B
MOV A, E
STAX B
HLT
9. Write an 8085 program to display the BCD digits from 0 to 9 the seven segments as in the following diagram. Use the activating data bits the same as the segment number as in the figure below. [2059 Shrawan]
LXI SP, 2999H
LXI H, 2050H
MOV M, 3FH
INX H
MOV M, 06H
INX H
MOV M, 5BH
INX H
MOV M, 4FH
INX H
MOV M, 66H
INX H
MOV M, 6DH
INX H
MOV M, 7DH
INX H
MOV M, 07H
INX H
MOV M, 7FH
INX H
MOV M, 6FH
LXI B, 2060H
LDAX B; Where the BCD digit is located
ANI F0H
RRC
RRC
RRC
RRC
CALL CODE
OUT PORT 1
LDAX B
ANI 0FH
CALL CODE
OUT PORT 2
HLT
CODE: LXI H, 2050H
ADD L
MOV L, A
MOV A, M
RET
HLT
10. Write a program for 8085 to change the bit D5 of ten numbers stored at address 7600H if the numbers are larger than or equal to 80H. [2061 Ashwin]
LXI H, 7600H
MVI C, 0AH
L2: MOV A, M
CPI 80H
JC L1
XRI 20H
MOV M, A
L1: INX H
DCR C
JNZ L2
You may also like Microprocessor 8085 programming Part 2
Leave a Reply