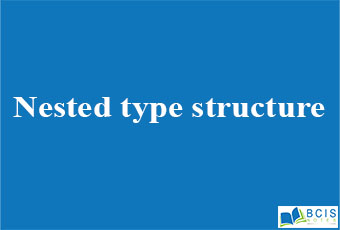
Nested type structure
Nested structure in C is nothing but the structure within a structure. One structure can be declared inside other structures as we declare structure members inside a structure.
The structure variables can be a normal structure variable or a pointer variable to access the data. You can learn the concepts in this section.
Nested structure in C is nothing but the structure within a structure. One structure can be declared inside other structures as we declare structure members inside a structure.
The structure variables can be a normal structure variable or a pointer variable to access the data. You can learn the concepts in this section.
1.Structure within the structure in C using normal variable
2.Structure within the structure in C using pointer variable
STRUCTURE WITHIN STRUCTURE IN C USING NORMAL VARIABLE:
This program explains how to use structure within the structure in C using normal variable. “student_college_detail’ structure is declared inside the “student_detail” structure in this program. Both structure variables are normal.
Please note that members of the “student_college_detail” structure are accessed by 2 dots (.) operator and members of the “student_detail” structure are accessed by a single dot(.) operator.
#include <stdio.h>
#include <string.h>
struct student_college_detail
{
int college_id;
char college_name[50];
};
struct student_detail
{
int id;
char name[20];
float percentage;
// structure within structure
struct student_college_detail clg_data;
}stu_data;
int main()
{
struct student_detail stu_data = {1, “Raju”, 90.5, 71145,
“Anna University”};
printf(” Id is: %d \n”, stu_data.id);
printf(” Name is: %s \n”, stu_data.name);
printf(” Percentage is: %f \n\n”, stu_data.percentage);
printf(” College Id is: %d \n”,
stu_data.clg_data.college_id);
printf(” College Name is: %s \n”,
stu_data.clg_data.college_name);
return 0;
}
OUTPUT:
Id is: 1
Name is: Raju
Percentage is: 90.500000
College Id is: 71145
College Name is: Anna University
STRUCTURE WITHIN STRUCTURE IN C USING POINTER VARIABLE:
This program explains how to use structure within the structure in C using the pointer variable. “student_college_detail’ structure is declared inside the “student_detail” structure in this program. one normal structure variable and one pointer structure variable is used in this program.
Please note that a combination of. (dot) and ->(arrow) operators are used to access the structure member which is declared inside the structure.
#include <stdio.h>
#include <string.h>
struct student_college_detail
{
int college_id;
char college_name[50];
};
struct student_detail
{
int id;
char name[20];
float percentage;
// structure within structure
struct student_college_detail clg_data;
}stu_data, *stu_data_ptr;
int main()
{
struct student_detail stu_data = {1, “Raju”, 90.5, 71145,
“Anna University”};
stu_data_ptr = &stu_data;
printf(” Id is: %d \n”, stu_data_ptr->id);
printf(” Name is: %s \n”, stu_data_ptr->name);
printf(” Percentage is: %f \n\n”,
stu_data_ptr->percentage);
printf(” College Id is: %d \n”,
stu_data_ptr->clg_data.college_id);
printf(” College Name is: %s \n”,
stu_data_ptr->clg_data.college_name);
return 0;
}
OUTPUT:
Id is: 1
Name is: Raju
Percentage is: 90.500000
College Id is: 71145
College Name is: Anna University
These are then conceptual notes of Nested type structure
You may also like: Generations of programming language
Leave a Reply