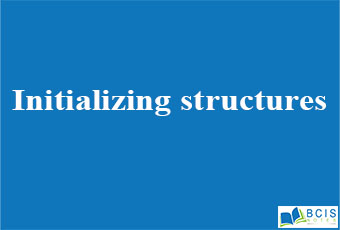
Initializing structures
The structure is used for collecting different data types together. The structure is Collection of different data types. There are different ways of calculating the size of the structure.
Different ways of calculating the size of the structure
1.By observation
2.By Using sizeof Operator
3.Without using Sizeof Operator
Way 1 : Calculate by adding Individual Sizes
struct Book
{
int pages;
char name[10];
char author[10];
float price;
}b1;
Calculating Size of Structure :
Size = size of ‘Pages’ + size of ‘Name’ +
size of ‘Author’ + size of ‘Price’
= 2 + 10 * 1 + 10 * 1 + 4
= 2 + 10 + 10 + 4
= 26
Important Note :
- Add all the individual Size of Each Structure Member .
- Character array requires Memory Equivalent to (No_of_elements * Sizeof(char))
- Float, Integer requires 4,2 bytes respectively in Case of Borland CC++ 3.0
Way 2: Using Sizeof Operator
Sizeof Operator is used to Calculating the Size of data type, variable, expression result. Syntax of sizeof is much like function, but sizeof is not a library function. Sizeof is C Operator.
#include<stdio.h>
typedef struct b1 {
char bname[30];
int ssn;
int pages;
}book;
book b1 = {“Let Us C”,”1000″,90};
int main()
{
printf(“\nSize of Structure : %d”,sizeof(b1));
return(0);
}
Output :
Size of Structure : 26
Way3: Without Using Sizeof Operator
#include<stdio.h>
struct
{
int a1,a2;
char s1;
int *ptr;
int arr[5];
}a[2];
void main()
{
int start,last;
start = &a[1].a1;
last = &a[0].a1;
printf(“\nSize of Structure : %d Bytes”,start-last);
}
We can evaluate size of structure without using sizeof operator. We can find difference between them to find size of structure.
These are the conceptual notes of Initializing structures
You may also like: Program design methodology
Leave a Reply