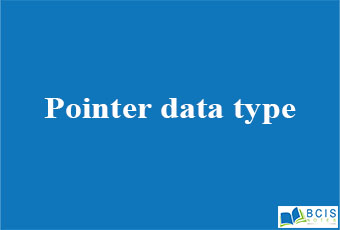
Pointer data type
The pointer data type is unique among the FreeBasic numeric data types. Instead of containing data, like the other numeric types, a pointer contains the memory address of data.
On a 32-bit system, the pointer data type is 4 bytes. FreeBasic uses pointers for several functions such as ImageCreate, and pointers are used heavily in external libraries such as the Windows API. Pointers are also quite fast since the compiler can directly access the memory location that a pointer points to. A proper understanding of pointers is essential to effective programming in FreeBasic.
For many beginning programmers, pointers seem like a strange and mysterious beast. However, if you keep one rule in mind, you should not have any problems using pointers in your program. The rule is very simple: a pointer contains an address, not data. If you keep this simple rule in mind, you should have no problems using pointers.
Pointers and Memory
You can think of the memory in your computer as a set of post office boxes (P.O. Box) at your local post office. When you go in to rent a P.O. Box, the clerk will give you a number, such as 100. This is the address of your P.O. Box. You decide to write the number down an a slip of paper and put it in your wallet. The next day you go to the post office and pull out the slip of paper. You locate box 100 and look inside the box and find a nice stack of junk mail. Of course, you want to toss the junk mail, but there isn’t a trash can handy, so you decide to just put the mail back in the box and toss it later. Working with pointers in FreeBasic is very similar to using a P.O. Box.
When you declare a pointer, it isn’t pointing to anything analogous to the blank slip of paper. To use a pointer, it must be initialized to a memory address, which is the same as writing down the number 100 on the slip of paper. Once you have the address, find the right P.O. Box, you can dereference the pointer, open the mailbox, add or retrieve data from the pointed-to memory location. As you can see there are three basic steps to using pointers.
1. Declare a pointer variable.
2. Initialize the pointer to a memory address.
3. Dereference the pointer to manipulate the data at the pointed-to memory location.
This isn’t any different than using a standard variable, and you use pointers in much the same way as standard variables. The only real difference between the two is that in a standard variable, you can access the data directly, and with a pointer, you must dereference the pointer to interact with the data.
Typed and Untyped Pointers
FreeBasic has two types of pointers, typed and untyped. A typed pointer is declared with an associated data type.
Dim pointer as Integer Ptr
This tells the compiler that this pointer will be used for integer data. Using typed pointers allows the compiler to do type checking to make sure that you are not using the wrong type of data with the pointer, and simplifies pointer arithmetic.
Untyped pointers are declared using the Any keyword.
Dim pointer as Any Ptr
Untyped pointers have no type checking and default to the size of a byte. Untyped pointers are used in the C Runtime Library and many third-party libraries, such as the Win32 API, to accommodate the void pointer type in C. Unless you specifically need an untyped pointer, you should use typed pointers so that the compiler can check the pointer assignments.
You may also like: Debugging a Program
Leave a Reply