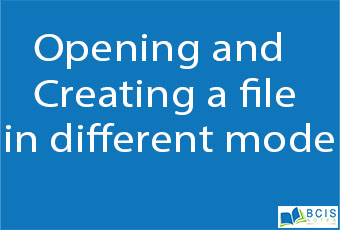
Opening and Creating a file in different modes
C File management
A File can be used to store a large volume of persistent data. Like many other languages ‘C’ provides following file management functions,
- Creation of a file
- Opening a file
- Reading a file
- Writing to a file
- Closing a file
Following are the most important file management functions available in ‘C,’
function purpose
fopen () Creating a file or opening an existing file
fclose () Closing a file
fprintf () Writing a block of data to a file
fscanf () Reading a block data from a file
getc () Reads a single character from a file
puts () writes a single character to a file
get () Reads an integer from a file
puts () Writing an integer to a file
fseek () Sets the position of a file pointer to a specified location
ftell () Returns the current position of a file pointer
rewind () Sets the file pointer at the beginning of a file
In this tutorial, you will learn-
How to Create a File
How to Close a file:
Writing to a File
fputc() Function:
fputs () Function:
fprintf()Function:
Reading data from a File
Interactive File Read and Write with getc and put
How to Create a File
Whenever you want to work with a file, the first step is to create a file. A file is nothing but space in a memory where data is stored.
To create a file in a ‘C’ program following syntax is used,
FILE *fp;
fp = fopen (“file_name”, “mode”);
In the above syntax, the file is a data structure which is defined in the standard library.
fopen is a standard function which is used to open a file.
If the file is not present on the system, then it is created and then opened.
If a file is already present on the system, then it is directly opened using this function.
fp is a file pointer which points to the type file.
Whenever you open or create a file, you have to specify what you are going to do with the file. A file in ‘C’ programming can be created or opened for reading/writing purposes. A mode is used to specify whether you want to open a file for any of the below-given purposes. Following are the different types of modes in ‘C’ programming which can be used while working with a file.
File Mode Description
r Open a file for reading. If a file is in reading mode, then no data is deleted if a file is already present on a system.
w Open a file for writing. If a file is in writing mode, then a new file is created if a file doesn’t exist at all. If a file is already present on a system, then all the data inside the file is truncated, and it is opened for writing purposes.
an Open a file in append mode. If a file is in append mode, then the file is opened. The content within the file doesn’t change.
r+ Open for reading and writing from beginning
w+ Open for reading and writing, overwriting a file
a+ Open for reading and writing, appending to file
In the given syntax, the filename and the mode are specified as strings hence they must always be enclosed within double-quotes.
Example:
#include <stdio.h>
int main() {
FILE *fp;
fp = fopen (“data.txt”, “w”);
}
Output:
File is created in the same folder where you have saved your code.
These are the conceptual notes of Opening and Creating a file in different modes
You may also like: History of Computing and Computers
Leave a Reply