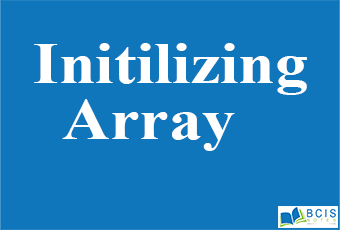
Arrays
Arrays and Strings allow for a set of variables to be grouped as one variable. This is useful in its own right, but also because strings fall into this category. Strings, which are how we represent words and sentences in computer languages, are just collections of character variables. Therefore, we represent strings using arrays in C.
we didn’t bother specifying a number in the square brackets. This is because the curly brackets have values in them that will be assigned to each position in the array. You could put a number in the brackets anyway, as long as you made sure to create enough memory locations to store the values you’ve passing in.
float my_array[5] = {5.0, 2.5};
If you partially initialize an array, the compiler sets the remaining elements to zero.
Now that the array has been declared with 5 values, it has 5 memory locations. Consider this table for a visual example of that:
Position 0 1 2 3 4
Value 1 5 3 6 2
Any integer can be placed in the square brackets to get a position in the array. Those integers can be variables, too. Take a look at this example, which prints the contents of an array:
#include <stdio.h>
int main(void) {
int my_array[] = {1, 1, 2, 3, 5, 7, 12};
for(int count = 0; count < 7; count++) {
printf(“%i, \n”, my_array[count]);
}
return 0;
}
How to initialize an array in C programming?
It’s possible to initialize an array during declaration. For example,
int mark[5] = {19, 10, 8, 17, 9};
Another method to initialize array during declaration:
int mark[] = {19, 10, 8, 17, 9};
You may also like: History of Computing and Computers
Leave a Reply